Universal Feed
Overview
UniversalFeed
displays a universal feed screen with a topic selection bar and a list of posts, create post button.
GitHub files
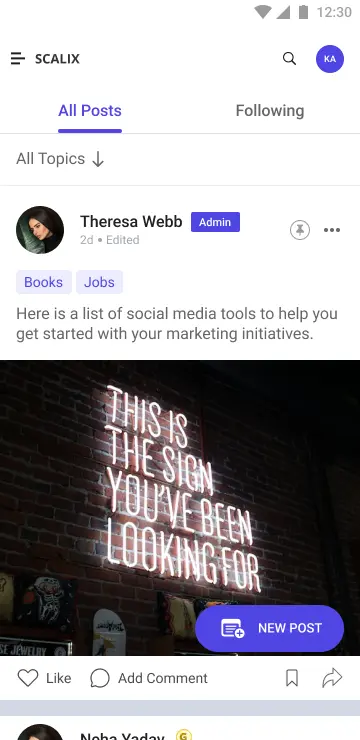
UI Components
The Universal Feed screen serves as a parent component, allowing you to incorporate as many child components as necessary, including your own custom components. Here are a few components that can be utilized to enhance the universal feed.
Props
These props allow interaction handling and feature customization for the UniversalFeed
component:
Interaction Callbacks
Prop Name | Type | Description |
---|---|---|
postLikeHandlerProp | Function | Triggered when a post is liked. Receives the post id . |
savePostHandlerProp | Function | Triggered when a post is saved or unsaved. Receives id and saved . |
selectPinPostProp | Function | Triggered to pin or unpin a post. Receives id and pinned status. |
selectEditPostProp | Function | Allows editing of a post. Receives id and post data. |
onSelectCommentCountProp | Function | Triggered when the comment count is tapped. Receives post id . |
onTapLikeCountProps | Function | Triggered when the like count is tapped. Receives post id . |
handleDeletePostProps | Function | Allows deletion of a post. Receives visible and postId . |
handleReportPostProps | Function | Handles reporting of a post. Receives post id . |
newPostButtonClickProps | Function | Custom action for the new post button. |
onOverlayMenuClickProp | Function | Custom action for overlay menu clicks. Receives event, menu items, post id . |
onTapNotificationBellProp | Function | Triggered when the notification bell is tapped. |
onSharePostClicked | Function | Handles sharing of a post. Receives post id . |
onSubmitButtonClicked | Function | Custom action for poll submission. |
onAddPollOptionsClicked | Function | Custom action for adding poll options. |
onPollOptionClicked | Function | Triggered when a poll option is clicked. |
onCancelPressProp | Function | Action when cancel button is pressed for upload retry. |
onRetryPressProp | Function | Action when retry button is pressed for upload retry. |
onSearchIconClickProp | Function | Action triggered when the search icon is clicked. |
Feature Configuration
Prop Name | Type | Description | Required |
---|---|---|---|
isHeadingEnabled | boolean | Enables or disables the heading display. | ✅ |
isTopResponse | boolean | Shows the top response if true. | |
hideTopicsView | boolean | Hides the topic selection view in the feed. |
Styling Customizations
The STYLES
class allows appearance customization for the UniversalFeed
via universalFeedStyle
.
Style Prop | Type | Description |
---|---|---|
newPostButtonStyle | ViewStyle | Style for the new post button. |
newPostButtonText | TextStyle | Style for the new post button text. |
newPostIcon | LMImageProps | Icon used in the new post button. |
screenHeader | LMHeaderProps | Style and props for the screen header. |
Usage Example
Step 1: Create the Feed Screen Component
- Create a
CustomFeedScreen
file to define your custom feed logic and UI using hooks and context from the LikeMinds Feed SDK.
import React, { useEffect, useState } from "react";
import {
LMCreatePostButton,
LMFilterTopics,
LMPostUploadIndicator,
LMUniversalFeedHeader,
PostsList,
UniversalFeed,
usePostListContext,
useUniversalFeedContext,
} from "@likeminds.community/feed-rn-core";
import { Alert, Platform, Share } from "react-native";
import { useAppSelector } from "@likeminds.community/feed-rn-core/store/store";
import STYLES from "@likeminds.community/feed-rn-core/constants/Styles";
import { useNavigation } from "@react-navigation/native";
const CustomFeedScreen = () => {
const {
handleEditPost,
handlePinPost,
} = usePostListContext();
const mappedTopics = useAppSelector((state) => state.feed.mappedTopics);
const navigation = useNavigation();
const customHandleEdit = (postId, post) => {
console.log("before edit select", post);
handleEditPost(postId, post);
console.log("after edit select", postId);
};
const customHandlePin = (postId, pinned) => {
console.log("before pin select");
handlePinPost(postId, pinned);
console.log("after pin select", postId, pinned);
};
const universaleFeedStyles = {
newPostButtonStyle: {
backgroundColor: "red",
border: 1,
},
screenHeader: {
heading: "LikeMinds",
subHeading: "Welcome to LM Documentation",
onBackPress: () => navigation.goBack(),
},
};
// universal feed screen customisation
if (universaleFeedStyles) {
STYLES.setUniversalFeedStyles(universaleFeedStyles);
}
return (
<UniversalFeed
selectEditPostProp={(id, post) => customHandleEdit(id, post)}
selectPinPostProp={(id, pinned) => customHandlePin(id, pinned)}
isHeadingEnabled={true}
isTopResponse={true}
>
<LMUniversalFeedHeader />
<LMFilterTopics />
<LMPostUploadIndicator />
<PostsList items={mappedTopics} />
<LMCreatePostButton />
</UniversalFeed>
);
};
export default CustomFeedScreen;
Step 2: Wrap the Screen with Context Providers
- Create a
FeedWrapper
component that wraps your custom feed screen withUniversalFeedContextProvider
andPostListContextProvider
.
import CustomFeedScreen from "<<path_to_FeedScreen.tsx>>";
import {
PostListContextProvider,
UniversalFeedContextProvider,
} from "@likeminds.community/feed-rn-core";
const FeedWrapper = ({ navigation, route }) => {
return (
<UniversalFeedContextProvider navigation={navigation} route={route}>
<PostListContextProvider navigation={navigation} route={route}>
<CustomFeedScreen />
</PostListContextProvider>
</UniversalFeedContextProvider>
);
};
export default FeedWrapper;
Step 3: Add the Custom Screen in App.tsx
- In your
App.tsx
, create aStack.Navigator
in theNavigationContainer
wrapped byLMOverlayProvider
. - Add
FeedWrapper
as a Stack screen in yourNavigationContainer
.
import {
UNIVERSAL_FEED,
LMOverlayProvider,
} from "@likeminds.community/feed-rn-core";
import { NavigationContainer } from "@react-navigation/native";
import { createNativeStackNavigator } from "@react-navigation/native-stack";
import { FeedWrapper } from "<<path_to_CustomisedUniversalFeed.tsx>>";
export const App = () => {
const Stack = createNativeStackNavigator();
return (
<LMOverlayProvider
myClient={myClient} // pass in the LMFeedClient created
apiKey={apiKey} // pass in the API Key generated
userName={userName} // pass in the logged-in user's name
userUniqueId={userUniqueID} // pass in the logged-in user's uuid
>
<NavigationContainer ref={navigationRef} independent={true}>
<Stack.Navigator screenOptions={{ headerShown: true }}>
<Stack.Screen name={UNIVERSAL_FEED} component={FeedWrapper} />
</Stack.Navigator>
</NavigationContainer>
</LMOverlayProvider>
);
};