Notification Feed
Overview
The LMFeedNotificationFeedScreen
is designed to present a list of notifications to the user. Users can pull to refresh the list of notifications, and the screen shows an empty state view when there are no notifications to display. It supports navigating to different parts of the app based on the notification's content.
GitHub Files:
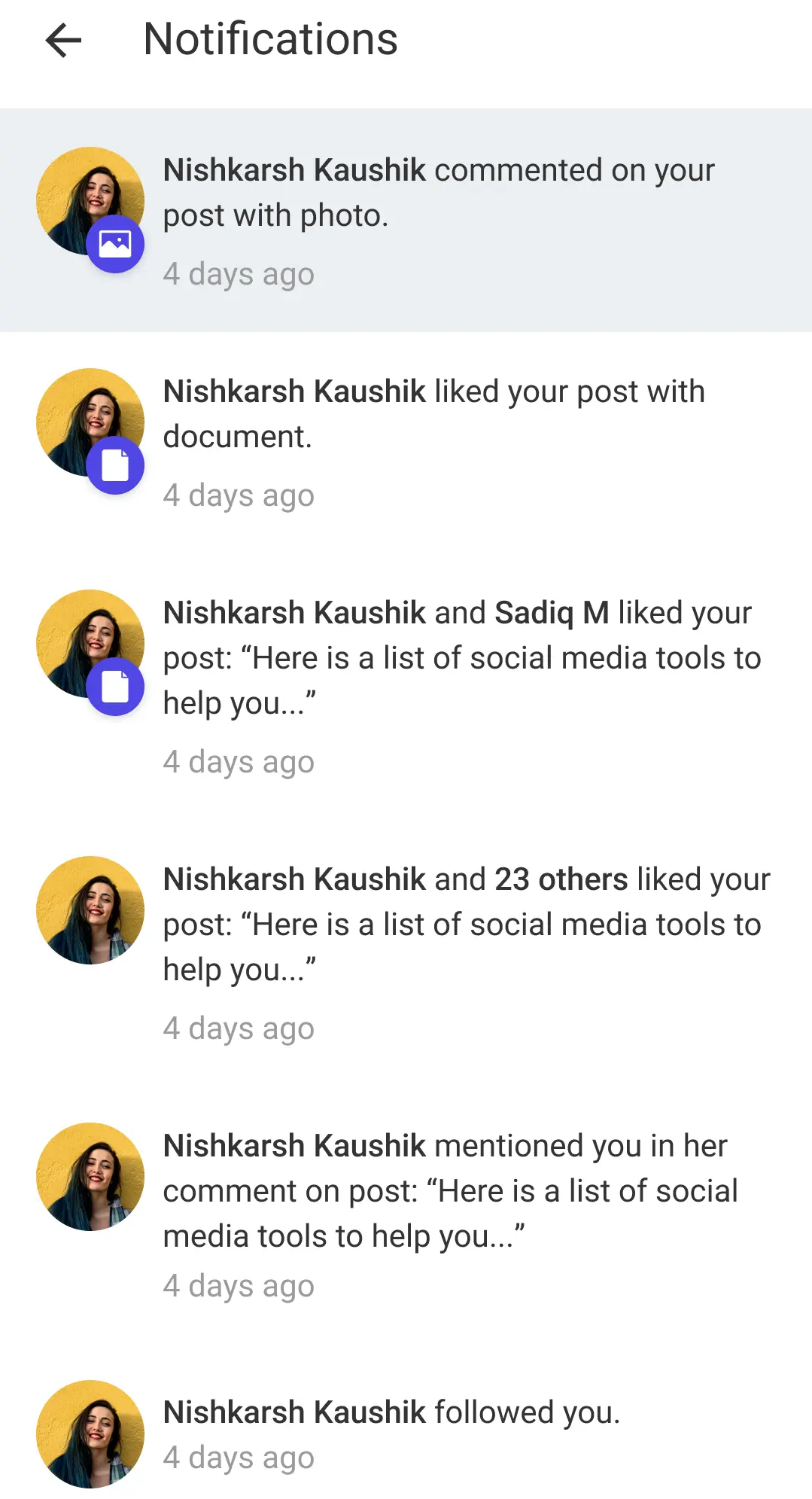
UI Components
Props
These props allow interaction handling and functional customization for the NotificationFeed
component:
Interaction Callbacks
Property | Type | Description |
---|---|---|
onNotificationItemClickedProp | Function | Triggered when a notification item is clicked. Provides LMActivityViewData . |
Styling Customizations
The STYLES
class allows you to customize the appearance of the Notification Feed. You can set styles using notificationFeedStyle
in STYLES
.
Customization Table
Style Prop | Type | Description |
---|---|---|
screenHeader | LMHeaderProps | Customizes the screen header. |
backgroundColor | string | Background color of the screen. |
unreadBackgroundColor | string | Background color for unread notifications. |
activityTextStyles | TextStyle | Styles for the activity text. |
timestampTextStyles | TextStyle | Styles for the timestamp text. |
userImageStyles | UserImageStyles | Styles for user image, including fallback and size. |
activityAttachmentImageStyle | LMIconProps | Style for activity attachment image. |
noActivityViewText | string | Text displayed when there are no activities. |
noActivityViewTextStyle | TextStyle | Style for the no activity text. |
noActivityViewImage | React.ReactNode | Image shown when there are no activities. |
noActivityViewImageStyle | ImageStyle | Style for the no activity image. |
customScreenHeader | React.ReactNode | Custom component to replace the default screen header. |
activityTextComponent | Function | Custom component for rendering activity text. |
UserImageStyles
Property | Type | Description |
---|---|---|
fallbackTextStyle | TextStyle | Styles for fallback text if the image fails to load. |
size | number | Size of the user image. |
onTap | Function | Callback when the user image is tapped. |
fallbackTextBoxStyle | ViewStyle | Style for the fallback text container. |
profilePictureStyle | ImageStyle | Style for the user profile picture. |
Usage Example
Step 1: Create the Notification Screen Component
- Define the
CustomNotificationScreen
component, where you customize callback behavior and apply styling.
import {
LMFeedNotificationFeedScreen,
useNotificationFeedContext,
} from "@likeminds.community/feed-rn-core";
const CustomNotificationScreen = () => {
const { handleActivityOnTap, handleScreenBackPress } =
useNotificationFeedContext();
// customised handleActivityOnTap callback
const customNotificationOnTap = (activity) => {
console.log("do something before notification tap", activity);
handleActivityOnTap(activity);
console.log("do something after notification tap");
};
const notificationFeedStyles = {
backgroundColor: "green",
unreadBackgroundColor: "red",
};
// notification feed screen customisation
if (notificationFeedStyles) {
STYLES.setNotificationFeedStyles(notificationFeedStyles);
}
return (
<LMFeedNotificationFeedScreen
onNotificationItemClickedProp={(activity) =>
customNotificationOnTap(activity)
}
/>
);
};
export default CustomNotificationScreen;
Step 2: Wrap the Screen with Context Provider
- Create a
NotificationScreenWrapper
that wraps theCustomNotificationScreen
withNotificationFeedContextProvider
.
import { NotificationFeedContextProvider } from "@likeminds.community/feed-rn-core";
import CustomNotificationScreen from "<<path_to_NotificationScreen.tsx>>";
const NotificationScreenWrapper = ({ navigation, route }) => {
return (
<NotificationFeedContextProvider navigation={navigation} route={route}>
<CustomNotificationScreen />
</NotificationFeedContextProvider>
);
};
export default NotificationScreenWrapper;
Step 3: Add the Custom Screen in App.tsx
- In your
App.tsx
, create aStack.Navigator
in theNavigationContainer
wrapped byLMOverlayProvider
. - Add
NotificationScreenWrapper
as a Stack screen in yourNavigationContainer
.
import {
NOTIFICATION_FEED,
LMOverlayProvider,
STYLES,
} from "@likeminds.community/feed-rn-core";
import NotificationScreenWrapper from "<<path_to_NotificationScreenWrapper.tsx>>";
import { NavigationContainer } from "@react-navigation/native";
import { createNativeStackNavigator } from "@react-navigation/native-stack";
export const App = () => {
const Stack = createNativeStackNavigator();
return (
<LMOverlayProvider
myClient={myClient} // pass in the LMFeedClient created
apiKey={apiKey} // pass in the API Key generated
userName={userName} // pass in the logged-in user's name
userUniqueId={userUniqueID} // pass in the logged-in user's uuid
>
<NavigationContainer ref={navigationRef} independent={true}>
<Stack.Navigator screenOptions={{ headerShown: false }}>
<Stack.Screen
name={NOTIFICATION_FEED}
component={NotificationScreenWrapper}
/>
</Stack.Navigator>
</NavigationContainer>
</LMOverlayProvider>
);
};