Post List View
Overview
The PostsList
component is responsible for rendering a list of posts in a feed. It handles the display of individual post items and provides support for infinite scrolling, ensuring smooth loading of new content as the user navigates through the feed. The component integrates with post content, media, header, and footer, offering extensive customization options for styling and functionality.
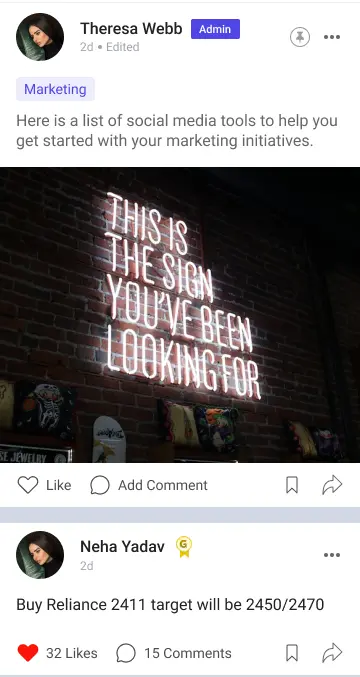
UI Components
Data Variables
feedData
: Stores the feed data conforming toLMPostViewData[]
.
Callbacks
postLikeHandlerProp
: Handles the action when a post is liked.savePostHandlerProp
: Handles the action when a post is saved or unsaved.selectPinPostProp
: Handles the action when a post is pinned or unpinned.
For information about more callbacks, click here.
Customization
The STYLES
class allows you to customize the appearance of the LMPostContent
. You can set the styles in postListStyle
in STYLES
.
Property | Type | Description |
---|---|---|
header | LMFeedHeaderProps | Customization for the header of the post. |
footer | LMFeedFooterProps | Customization for the footer of the post. |
postContent | LMFeedPostContentProps | Customization for the post content area. |
media | LMFeedMediaProps | Customization for media like images, videos, and documents in the post. |
noPostView | ViewStyle | Styling for the view when no post is available. |
noPostText | TextStyle | Styling for the text displayed when no post is available. |
listStyle | ViewStyle | Styling for the overall list view. |
Usage Example
The PostsList
screen can be used by wrapping it inside the UniversalFeed
screen, and the selected topcis can be passed as a prop to this screen, so that filtered posts are displayed. The customised callbacks for PostsList
screen are passed to UniversalFeed
screen and are internally accessed by PostsList
screen.
Step 1: Create Custom Screen and Wrapper
- Create a
FeedScreenWrapper
file which will wrap theFeedScreen
within theUniversalFeedContextProvider
andPostListContextProvider
so that the callbacks becomes accessible inside of theFeedScreen
. - Create
postListStyles
for customisation and call thesetPostListStyles
to set the customisation.
- FeedScreen
- FeedScreenWrapper
import { PostsList, UniversalFeed } from "@likeminds.community/feed-rn-core";
import { useAppSelector } from "@likeminds.community/feed-rn-core/store/store";
// here, mappedTopics are the selected topics according to which the posts will be shown
const mappedTopics = useAppSelector((state) => state.feed.mappedTopics);
export const SamplePostList = () => {
const { postLikeHandler, savePostHandler, handleEditPost } =
usePostListContext();
// customised postLikeHandler method
const customPostLike = (postId) => {
console.log("before like ");
postLikeHandler(postId);
console.log("after like", postId);
};
// customised savePostHandler method
const customPostSave = (postId, saved) => {
console.log("before save");
savePostHandler(postId, saved);
console.log("after save", postId, saved);
};
// customised handlePinPost method
const customHandlePin = (postId, pinned) => {
console.log("before pin select");
handlePinPost(postId, pinned);
console.log("after pin select", postId, pinned);
};
const postListStyles = {
header: {
profilePicture: {
size: 25,
fallbackTextBoxStyle: "ProfilePicture",
},
},
showMemberStateLabel: true,
};
// universal feed screen customisation
if (postListStyles) {
STYLES.setPostListStyles(postListStyles);
}
return (
<UniversalFeed
postLikeHandlerProp={(id) => customPostLike(id)}
savePostHandlerProp={(id, saved) => customPostSave(id, saved)}
selectPinPostProp={(id, pinned) => customHandlePin(id, pinned)}
>
<PostsList items={mappedTopics} />
</UniversalFeed>
);
};
import FeedScreen from "<<path_to_FeedScreen.tsx>>";
import {
PostListContextProvider,
UniversalFeedContextProvider,
} from "@likeminds.community/feed-rn-core";
const FeedWrapper = ({ navigation, route }) => {
return (
<UniversalFeedContextProvider navigation={navigation} route={route}>
<PostListContextProvider navigation={navigation} route={route}>
<FeedScreen />
</PostListContextProvider>
</UniversalFeedContextProvider>
);
};
export default FeedWrapper;
Step 2: Add the Custom Screen in App.tsx
- In your
App.tsx
, create aStack.Navigator
in theNavigationContainer
wrapped byLMOverlayProvider
. - Add
FeedWrapper
as a Stack screen in yourNavigationContainer
.
import {
UNIVERSAL_FEED,
LMOverlayProvider,
} from "@likeminds.community/feed-rn-core";
import { NavigationContainer } from "@react-navigation/native";
import { createNativeStackNavigator } from "@react-navigation/native-stack";
import { FeedWrapper } from "<<path_to_CustomisedUniversalFeed.tsx>>";
export const App = () => {
const Stack = createNativeStackNavigator();
return (
<LMOverlayProvider
myClient={myClient} // pass in the LMFeedClient created
apiKey={apiKey} // pass in the API Key generated
userName={userName} // pass in the logged-in user's name
userUniqueId={userUniqueID} // pass in the logged-in user's uuid
>
<NavigationContainer ref={navigationRef} independent={true}>
<Stack.Navigator screenOptions={{ headerShown: true }}>
<Stack.Screen name={UNIVERSAL_FEED} component={FeedWrapper} />
</Stack.Navigator>
</NavigationContainer>
</LMOverlayProvider>
);
};