Chatroom Screen
Overview
The ChatRoom
screen serves as the main interface for users to engage in conversations within a specific chatroom in the LikeMinds chat application. It allows users to send and receive messages, view chatroom details, and interact with various features such as polls, media, and reactions, providing a seamless chat experience.
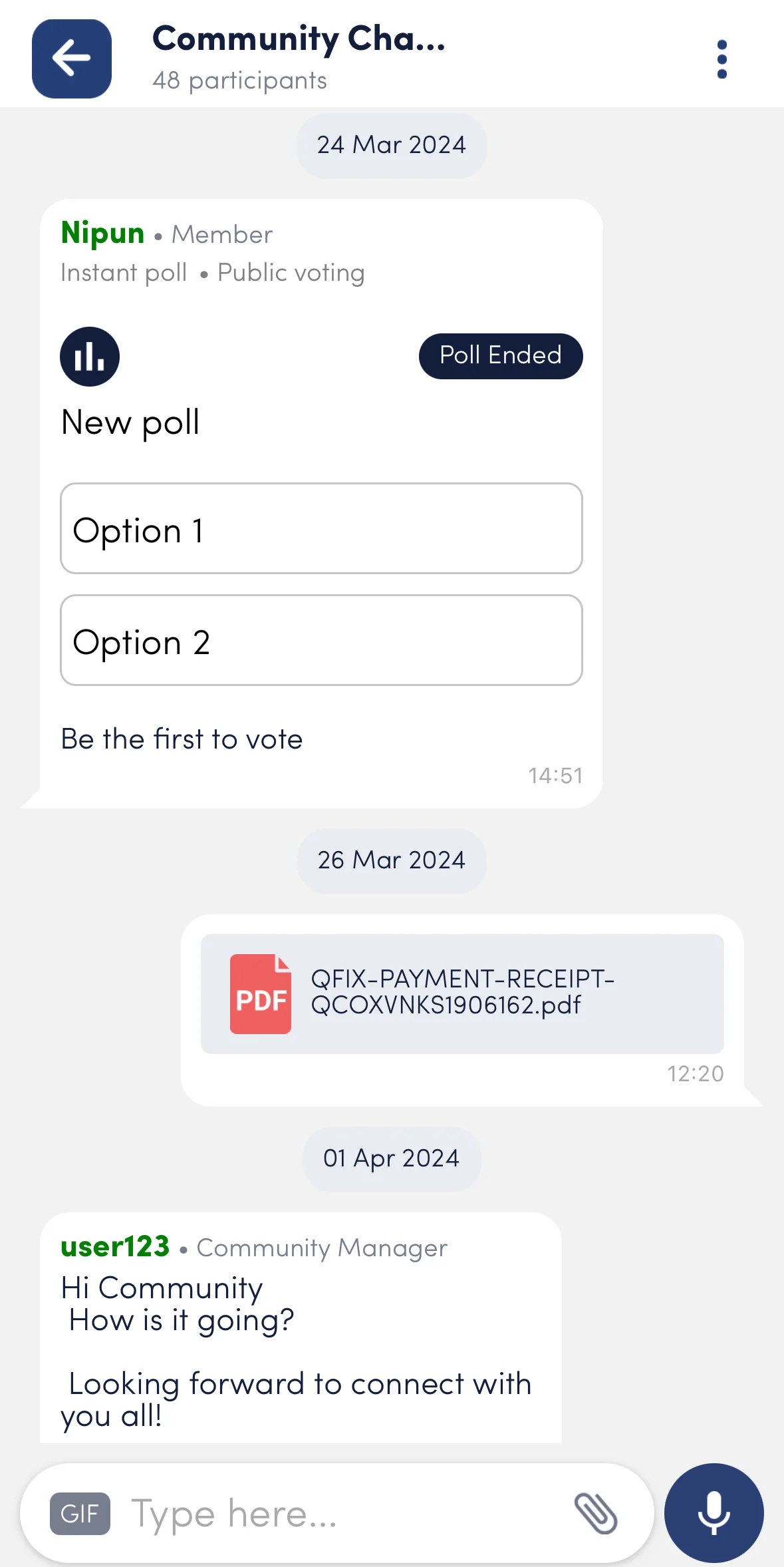
UI Components
The Chatroom screen serves as a parent component, allowing you to incorporate as many child components as necessary, including your own custom components. Here are a few components that can be utilized to enhance the Chatroom.
Callbacks
setChatroomTopic
: Triggered to set or update the chatroom's topic.leaveChatroom
: Triggered when the user opts to leave the chatroom.
For information about more callbacks, click here
Props
Property | Type | Description | Optional |
---|---|---|---|
children | ReactNode | The child components or content to render within the chatroom. | |
customReplyBox | Function | Custom function to render the reply box. | ✔️ |
customMessageHeader | ReactNode | Custom component for the message header. | ✔️ |
customMessageFooter | ReactNode | Custom component for the message footer. | ✔️ |
customVideoImageAttachmentConversation | ReactNode | Custom component for video and image attachments. | ✔️ |
For information about more props, click here
Usage Example
Step 1: Create Custom Screen and Wrapper
- In your app, create a
ChatroomScreenWrapper
file which will wrap theChatroomScreen
within theUniversalFeedContextProvider
andPostListContextProvider
so that the callbacks becomes accessible inside of theChatroomScreen
. - Now, you can create styles based on the component being utilizied, for example, for chatbubble create
messageListStyles
for customisation and call thesetMessageListStyles
to set it, orinputBoxStyle
for input box customisation and callsetInputBoxStyle
to set the customisation.
- ChatroomScreen
- ChatroomScreenWrapper
import {
ChatRoom,
ChatroomHeader,
MessageList,
MessageInput,
useChatroomContext,
useMessageListContext,
ChatroomTopic,
STYLES
} from "@likeminds.community/chat-rn-core";
import {InputBoxContextProvider} from '@likeminds.community/chat-rn-core/ChatSX/context/InputBoxContext';
import {ChatroomContextValues} from '@likeminds.community/chat-rn-core/ChatSX/context/ChatroomContext';
import MessageInputBox from "@likeminds.community/chat-rn-core/ChatSX/components/InputBox";
export function ChatroomScreen() {
const showViewParticipants = true;
const showShareChatroom = true;
const showChatroomIcon = true;
const customChatroomUserTitle = "Moderator";
const {
chatroomID,
chatroomWithUser,
currentChatroomTopic,
chatroomType,
replyChatID,
isEditable,
chatroomName,
isSecret,
refInput,
chatroomDBDetails,
chatRequestState,
setIsEditable,
handleFileUpload,
joinSecretChatroom,
onTapToUndo,
}: ChatroomContextValues = useChatroomContext();
const { scrollToBottom } = useMessageListContext();
const customJoinSecretChatroom = async () => {
console.log("before custom join secret chatroom");
const response = await joinSecretChatroom();
console.log("response after custom join secret chatroom", response);
};
const customOnTapToUndo = async () => {
console.log("before custom on tap to undo");
const response = await onTapToUndo();
console.log("response after custom on tap to undo", response);
};
const customScrollToBottom = async () => {
console.log("before custom scroll to bottom");
await scrollToBottom();
console.log("after custom scroll to bottom");
};
const inputBoxStyles = {
placeholderTextColor: "#aaa",
selectionColor: "#3CA874",
};
// custom styling for input box
if (inputBoxStyles) {
STYLES.setInputBoxStyle(inputBoxStyles);
}
const chatBubbleStyles = {
borderRadius: 10,
sentMessageBackgroundColor: 'blue',
};
// custom styling for message list
if (chatBubbleStyles) {
STYLES.setChatBubbleStyle(chatBubbleStyles);
}
return (
<ChatRoom
showViewParticipants={showViewParticipants}
showShareChatroom={showShareChatroom}
>
{/* ChatroomHeader */}
<ChatroomHeader
showChatroomIcon={showChatroomIcon}
customChatroomUserTitle={customChatroomUserTitle}
hideSearchIcon={true}
/>
{/* ChatroomTopic */}
<ChatroomTopic />
{/* MessageList */}
<MessageList
onTapToUndo={customOnTapToUndo}
scrollToBottom={customScrollToBottom}
/>
{/* Input Box Flow */}
<InputBoxContextProvider
chatroomName={chatroomName}
chatroomWithUser={chatroomWithUser}
replyChatID={replyChatID}
chatroomID={chatroomID}
isUploadScreen={false}
myRef={refInput}
handleFileUpload={handleFileUpload}
isEditable={isEditable}
setIsEditable={(value: boolean) => {
setIsEditable(value);
}}
isSecret={isSecret}
chatroomType={chatroomType}
currentChatroomTopic={currentChatroomTopic}
isPrivateMember={chatroomDBDetails.isPrivateMember}
chatRequestState={chatRequestState}>
<MessageInput joinSecretChatroomProp={customJoinSecretChatroom}>
<MessageInputBox />
</MessageInput>
</InputBoxContextProvider>
</ChatRoom>
);
}
import { Chat } from "@likeminds.community/chat-rn-core";
import { ChatroomScreen } from "<<path_to_ChatroomScreen.tsx>>";
function ChatroomScreenWrapper() {
return (
<Chat>
<ChatroomScreen />
</Chat>
);
}
export default ChatroomScreenWrapper;
Step 2: Add the Custom Screen in App.tsx
- In your
App.tsx
, create aStack.Navigator
in theNavigationContainer
wrapped byLMOverlayProvider
. - Add
ChatroomScreenWrapper
as a Stack screen in yourNavigationContainer
.
App.tsx
import { ScreenName, LMOverlayProvider, Themes } from "@likeminds.community/feed-rn-core";
import { NavigationContainer } from "@react-navigation/native";
import { createNativeStackNavigator } from "@react-navigation/native-stack";
import { ChatroomScreenWrapper } from "<<path_to_ChatroomScreenWrapper.tsx>>";
export const App = () => {
const Stack = createNativeStackNavigator();
return (
<LMOverlayProvider
myClient={myClient} // pass in the LMChatClient created
apiKey={apiKey} // pass in the API Key generated
userName={userName} // pass in the logged-in user's name
userUniqueId={userUniqueID} // pass in the logged-in user's uuid
theme={<"SDK_THEME">} // pass the sdk theme based on the Themes enum
>
<NavigationContainer ref={navigationRef} independent={true}>
<Stack.Navigator screenOptions={{ headerShown: true }}>
<Stack.Screen name={ScreenName.Chatroom} component={ChatroomScreenWrapper} />
</Stack.Navigator>
</NavigationContainer>
</LMOverlayProvider>
);
};