Search in Chatroom Screen
Overview
The SearchInChatroom
screen enables users to search for messages and content within a specific chatroom in the LikeMinds chat application. It provides a user-friendly interface for entering search queries and displays relevant results, making it easy for users to find specific discussions, media, or topics within the chat history. This feature enhances user experience by improving navigation and accessibility of past conversations.
GitHub File:
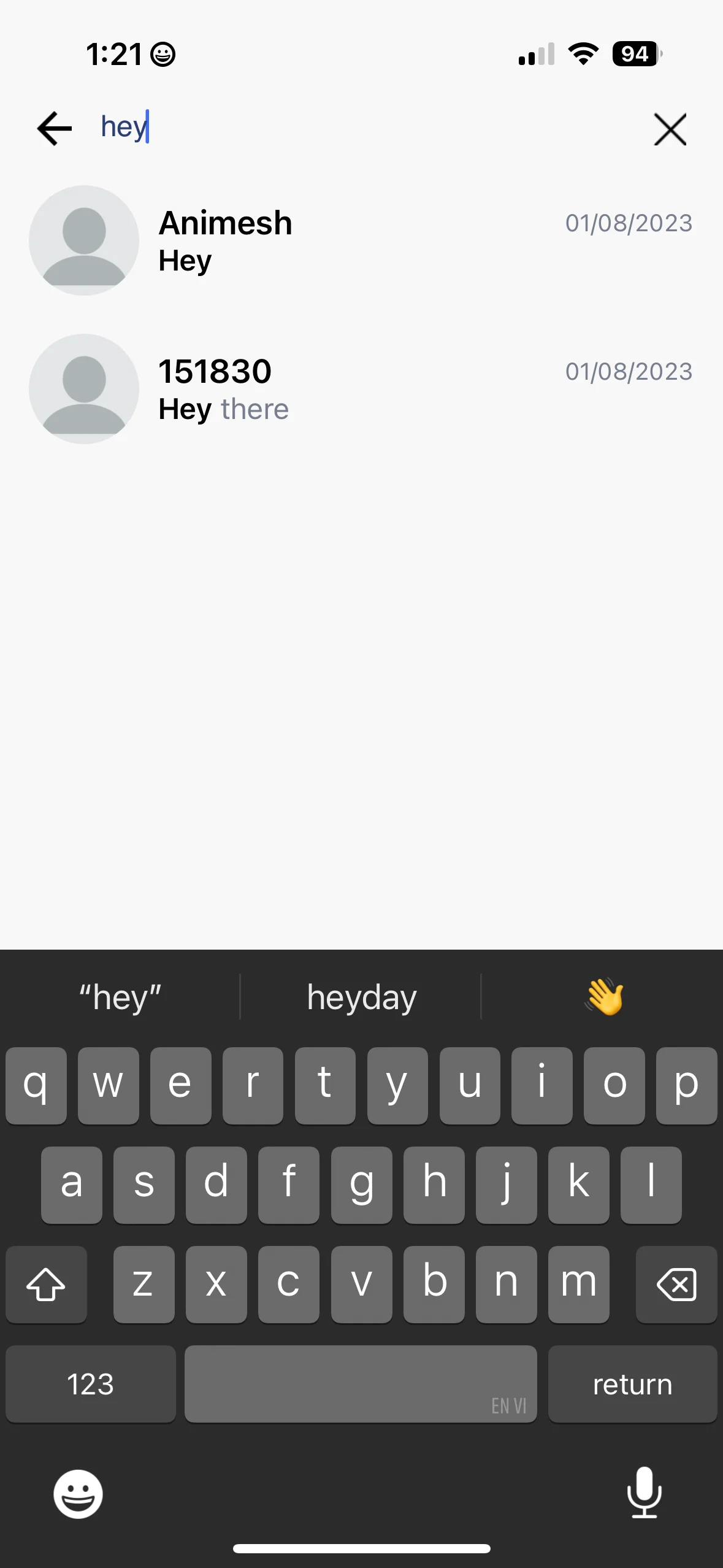
UI Components
Props
Customization Props
Property | Type | Description |
---|---|---|
customSearchHeader | Function | Custom function to render the search header. |
Styling Customisation
The SearchInChatroom
screen can be customised using the searchInChatroomStyles
Usage Example
Step 1: Create Custom Screen
- Create a
CustomSearchInChatroomScreen
file that defines the search screen and sets up styles and interaction callbacks as per requirement. - Create
searchInChatroomStyles
for customisation and call thesetSearchInChatroomStyle
to set the customisation.
import {
STYLES,
SearchInChatroomComponent,
useSearchInChatroomContext,
} from "@likeminds.community/chat-rn-core";
const CustomSearchInChatroomScreen = () => {
const { searchHeader } = useSearchInChatroomContext();
const searchInChatroomStyles = {
backArrowColor: "red",
crossIconColor: "blue",
searchPlaceholderTextColor: "Search...",
};
// custom styling for search in chatroom screen
if (searchInChatroomStyles) {
STYLES.setSearchInChatroomStyle(searchInChatroomStyles);
}
return <SearchInChatroomComponent customSearchHeader={ <CustomSearchHeader/> } />;
};
export default CustomSearchInChatroomScreen;
Step 2: Wrap the Screen with Context Provider
import { SearchInChatroomContextProvider } from "@likeminds.community/chat-rn-core";
import { SearchInChatroomScreen } from "<<path_to_CustomSearchInChatroomScreen.tsx>>";
function SearchInChatroomScreenWrapper() {
return (
<SearchInChatroomContextProvider>
<CustomSearchInChatroomScreen />
</SearchInChatroomContextProvider>
);
}
export default SearchInChatroomScreenWrapper;
Step 3: Add the Custom Screen in App.tsx
- In your
App.tsx
, create aStack.Navigator
in theNavigationContainer
wrapped byLMOverlayProvider
. - Add
SearchInChatroomScreenWrapper
as a Stack screen in yourNavigationContainer
.
App.tsx
import {
SEARCH_IN_CHATROOM,
LMOverlayProvider,
Themes
} from "@likeminds.community/feed-rn-core";
import { SearchInChatroomScreenWrapper } from "<<path_to_SearchInChatroomScreenWrapper.tsx>>";
import { NavigationContainer } from "@react-navigation/native";
import { createNativeStackNavigator } from "@react-navigation/native-stack";
export const App = () => {
const Stack = createNativeStackNavigator();
return (
<LMOverlayProvider
myClient={myClient} // pass in the LMFeedClient created
apiKey={apiKey} // pass in the API Key generated
userName={userName} // pass in the logged-in user's name
userUniqueId={userUniqueID} // pass in the logged-in user's uuid
theme={<"SDK_THEME">}
>
<NavigationContainer ref={navigationRef} independent={true}>
<Stack.Navigator screenOptions={{ headerShown: false }}>
<Stack.Screen
name={SEARCH_IN_CHATROOM}
component={SearchInChatroomScreenWrapper}
options={{
gestureEnabled: Platform.OS === "ios" ? false : true,
headerShown: false,
}}
/>
</Stack.Navigator>
</NavigationContainer>
</LMOverlayProvider>
);
};